While putting together my presentation for Boston Code Camp, I asked ChatGPT to help me create a Tic Tac Toe game. Using just three commands, I was able to generate a completely functioning web application.
Command #1
provide me the html, css, and javascript for a simple tic tac toe game. i’m using jquery and bootstrap. the game should start when the page loads. the player is X and always starts by clicking a square. the computer is O and continues the game until the game is over. if there is a tie, start the game over. include a scoreboard.
This command provided most of what I needed, including HTML, CSS, and JS code.
Tic Tac Toe
Player (X) Wins: 0
Computer (O) Wins: 0
.square {
cursor: pointer;
display: flex;
align-items: center;
justify-content: center;
font-size: 2rem;
}
$(document).ready(function() {
let player = "X";
let computer = "O";
let currentPlayer = player;
let playerWins = 0;
let computerWins = 0;
function checkWin() {
// Define your win logic here
// Return "Player" or "Computer" or "Tie" based on the board state
}
function computerMove() {
// Computer's move logic
// Find an empty square and mark it with 'O'
}
function resetGame() {
// Reset the game board for a new game
$('.square').text('');
currentPlayer = player;
}
$('.square').click(function() {
if ($(this).text() === '') {
$(this).text(currentPlayer);
let result = checkWin();
if (result) {
if (result === "Player") {
playerWins++;
$('#playerWins').text(playerWins);
} else if (result === "Computer") {
computerWins++;
$('#computerWins').text(computerWins);
}
setTimeout(resetGame, 2000);
} else {
currentPlayer = currentPlayer === player ? computer : player;
if (currentPlayer === computer) {
computerMove();
result = checkWin();
if (result) {
if (result === "Player") {
playerWins++;
$('#playerWins').text(playerWins);
} else if (result === "Computer") {
computerWins++;
$('#computerWins').text(computerWins);
}
setTimeout(resetGame, 2000);
} else {
currentPlayer = player;
}
}
}
}
});
});
Notice the JavaScript code includes comments that say things like “define logic here” without returning the full code. As if I have time for that! Let’s just ask for it…
Command #2
please provide me with the checkwin and computermove functions
I wasn’t sure if it would provide me with the code as expected. Why not just return those functions in full to begin with? That’s a question for another day. Sure enough, I was pleasantly surprised by the response. It even checks for a “tie.”
function checkWin() {
const squares = $('.square').map(function() { return $(this).text(); }).get();
const lines = [
[0, 1, 2], [3, 4, 5], [6, 7, 8], // Rows
[0, 3, 6], [1, 4, 7], [2, 5, 8], // Columns
[0, 4, 8], [2, 4, 6] // Diagonals
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] === squares[c]) {
return squares[a] === player ? "Player" : "Computer";
}
}
if (!squares.includes('')) {
return "Tie";
}
return null;
}
function computerMove() {
const emptySquares = $('.square').filter(function() {
return $(this).text() === '';
});
if (emptySquares.length > 0) {
const randomSquare = Math.floor(Math.random() * emptySquares.length);
emptySquares.eq(randomSquare).text(computer);
}
}
After taking the code and dropping it into a web app, here's what the site looks like. It actually works! While the computer move logic isn't perfect (it just picks a random empty square) the game has a back and forth mechanism that keeps track of wins and loses just like I asked. Super cool 😎
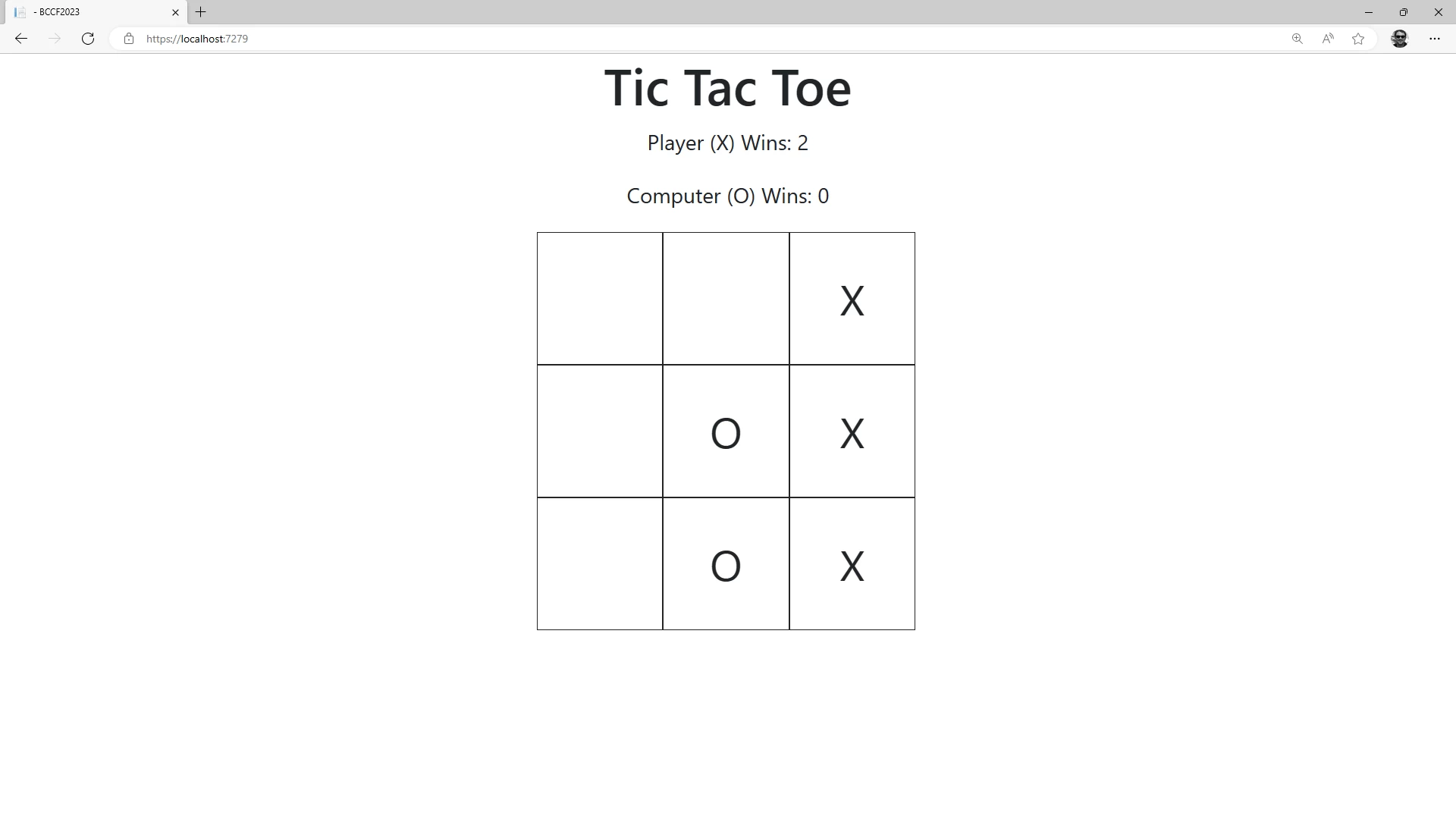
Looks pretty boring though. Let's spice it up with one more request.
Command #3
thanks! please add a bunch to the css so that this looks like a fun game. use lots of neon colors and gradients and use video game style fonts everywhere.
It's really neat trying out random things like this and actually having them work. Here's the CSS that was returned. The comments show you that the page will have a dark background, a retro video game font, and neon green and pink colors.
body {
background-color: #0d0d0d; /* Dark background */
color: white;
font-family: 'Press Start 2P', cursive; /* Retro video game font */
}
h1 {
color: #4dff4d; /* Neon green */
text-shadow: 0 0 10px #00ff00, 0 0 20px #00ff00, 0 0 30px #00ff00, 0 0 40px #00ff00, 0 0 70px #00ff00, 0 0 80px #00ff00, 0 0 100px #00ff00, 0 0 150px #00ff00;
}
#scoreboard p {
font-size: 1.2em;
color: #ff66ff; /* Neon pink */
text-shadow: 0 0 5px #ff00ff, 0 0 10px #ff00ff, 0 0 15px #ff00ff, 0 0 20px #ff00ff;
}
.square {
cursor: pointer;
display: flex;
align-items: center;
justify-content: center;
font-size: 2rem;
color: #00ccff; /* Neon blue */
background-image: linear-gradient(to right, #ff0080, #ff8c00, #40e0d0); /* Gradient */
border: 1px solid #ffffff; /* White border */
box-shadow: 0 0 5px #00ffff, 0 0 10px #00ffff, 0 0 15px #00ffff, 0 0 20px #00ffff; /* Neon glow */
}
.square:hover {
background-image: linear-gradient(to left, #ff0080, #ff8c00, #40e0d0); /* Inverse gradient on hover */
}
/* Import Google Font for Retro Video Game Style */
@import url('https://fonts.googleapis.com/css2?family=Press+Start+2P&display=swap');
ChatGPT instructs us to make sure the font import statement ends up where it needs to be. A quick move of that line to the top of the page and one refresh later, here's what our new Tic Tac Toe game looks like. AMAZING!
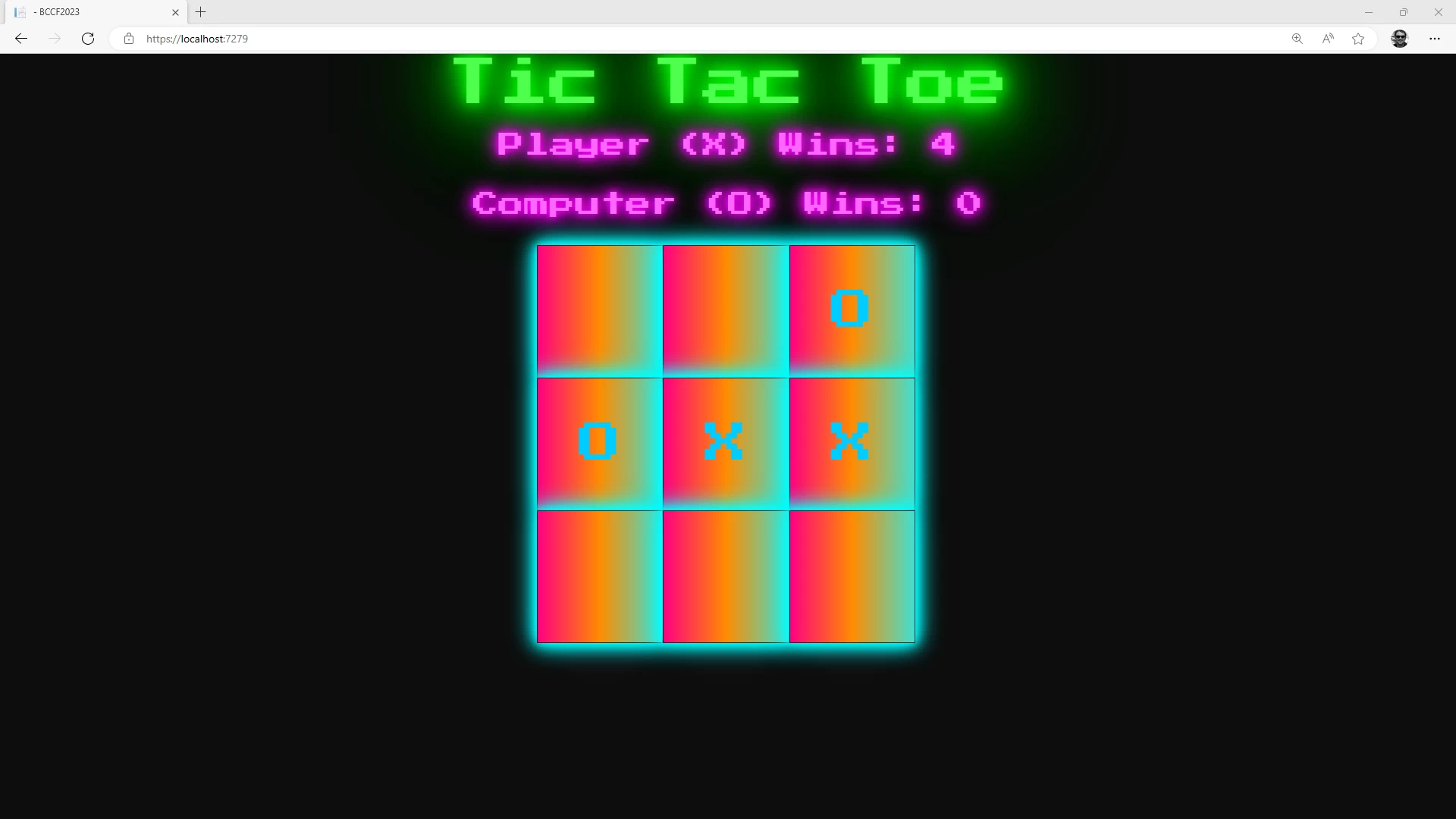